EFIxIDM (Ion drifts & effective masses)#
Abstract: Access to the
EFIxIDM
products from the SLIDEM project.
See also:
More notebooks: https://nbviewer.org/github/pacesm/jupyter_notebooks/tree/master/EFI_TII/EFIxIDM_00_data_access.ipynb
Product documentation: https://earth.esa.int/eogateway/documents/20142/2860886/SLIDEM_Product_Definition.pdf
SERVER_URL = 'https://vires.services/ows'
# Display important package versions used
%load_ext watermark
%watermark -i -v -p viresclient,pandas,xarray,matplotlib
Python implementation: CPython
Python version : 3.11.6
IPython version : 8.18.0
viresclient: 0.12.0
pandas : 2.1.3
xarray : 2023.12.0
matplotlib : 3.8.2
import datetime as dt
import matplotlib as mpl
import matplotlib.pyplot as plt
import numpy as np
import xarray as xr
# Control the HTML display of the datasets
xr.set_options(display_expand_attrs=False, display_expand_coords=True, display_expand_data=True)
from viresclient import SwarmRequest
request = SwarmRequest(SERVER_URL)
What data is available?#
request.available_collections("EFI_IDM", details=False)
{'EFI_IDM': ['SW_PREL_EFIAIDM_2_', 'SW_PREL_EFIBIDM_2_', 'SW_PREL_EFICIDM_2_']}
print(request.available_measurements("EFI_IDM"))
['Latitude_GD', 'Longitude_GD', 'Height_GD', 'Radius_GC', 'Latitude_QD', 'MLT_QD', 'V_sat_nec', 'M_i_eff', 'M_i_eff_err', 'M_i_eff_Flags', 'M_i_eff_tbt_model', 'V_i', 'V_i_err', 'V_i_Flags', 'V_i_raw', 'N_i', 'N_i_err', 'N_i_Flags', 'A_fp', 'R_p', 'T_e', 'Phi_sc']
Notes for the listed available variables:
idm_vars = [
# Positional information in geodetic (GD) and geocentric (GC) frames
# redundant with VirES variables Latitude, Longitude, Radius (in geocentric frame)
"Latitude_GD", "Longitude_GD", "Height_GD", "Radius_GC",
# Quasi-dipole magnetic latitude and local time
# redundant with VirES auxiliaries, QDLat & MLT
"Latitude_QD", "MLT_QD",
# Satellite velocity in NEC frame
"V_sat_nec",
# Estimated ion effective mass, uncertainty, and validity flags
"M_i_eff", "M_i_eff_err", "M_i_eff_Flags",
# Effective masses from ruhlik et al. (2015) topside empirical model
"M_i_eff_tbt_model",
# Along-track ion drift velocity, uncertainty, validity flags, and velocity without detrending
"V_i", "V_i_err", "V_i_Flags", "V_i_raw",
# Ion density, uncertainty, and validity flags
"N_i", "N_i_err", "N_i_Flags",
# Modified-OML faceplate area, and Langmuir spherical probe radius
"A_fp", "R_p",
# Electron temperature, and spacecraft floating potential
"T_e", "Phi_sc",
]
Fetching and plotting data#
start = dt.datetime(2016, 1, 2)
end = dt.datetime(2016, 1, 3)
request = SwarmRequest(SERVER_URL)
request.set_collection("SW_PREL_EFIAIDM_2_")
request.set_products(
measurements=idm_vars,
auxiliaries=["OrbitNumber", "OrbitDirection", "MLT"]
)
data = request.get_between(start, end)
Data can be loaded as either a pandas dataframe or a xarray dataset.
df = data.as_dataframe()
df.head()
V_i | M_i_eff_tbt_model | Phi_sc | Longitude | Longitude_GD | M_i_eff_err | Latitude | N_i_Flags | MLT | Radius_GC | ... | R_p | Latitude_GD | A_fp | OrbitDirection | N_i_err | V_i_Flags | OrbitNumber | M_i_eff_Flags | Spacecraft | V_i_err | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | |||||||||||||||||||||
2016-01-02 00:00:00.196999936 | -100000.0 | -1.0 | -2.263921 | -95.369735 | -95.369735 | -1.0 | 31.751028 | 1 | 17.155891 | 6.821611e+06 | ... | -1.0 | 31.912074 | -1.0 | 1 | -1.0 | 65537 | 11819 | 1 | A | -1.0 |
2016-01-02 00:00:00.696000000 | -100000.0 | -1.0 | -2.267120 | -95.369768 | -95.369768 | -1.0 | 31.783018 | 1 | 17.155951 | 6.821616e+06 | ... | -1.0 | 31.944152 | -1.0 | 1 | -1.0 | 65537 | 11819 | 1 | A | -1.0 |
2016-01-02 00:00:01.196999936 | -100000.0 | -1.0 | -2.271875 | -95.369800 | -95.369800 | -1.0 | 31.815135 | 1 | 17.156012 | 6.821621e+06 | ... | -1.0 | 31.976359 | -1.0 | 1 | -1.0 | 65537 | 11819 | 1 | A | -1.0 |
2016-01-02 00:00:01.696000000 | -100000.0 | -1.0 | -2.277763 | -95.369831 | -95.369831 | -1.0 | 31.847124 | 1 | 17.156071 | 6.821626e+06 | ... | -1.0 | 32.008436 | -1.0 | 1 | -1.0 | 65537 | 11819 | 1 | A | -1.0 |
2016-01-02 00:00:02.196999936 | -100000.0 | -1.0 | -2.265846 | -95.369860 | -95.369860 | -1.0 | 31.879242 | 1 | 17.156130 | 6.821630e+06 | ... | -1.0 | 32.040643 | -1.0 | 1 | -1.0 | 65537 | 11819 | 1 | A | -1.0 |
5 rows × 29 columns
ds = data.as_xarray()
ds
<xarray.Dataset> Dimensions: (Timestamp: 171434, V_sat_nec_dim1: 3) Coordinates: * Timestamp (Timestamp) datetime64[ns] 2016-01-02T00:00:00.1969999... Dimensions without coordinates: V_sat_nec_dim1 Data variables: (12/29) Spacecraft (Timestamp) object 'A' 'A' 'A' 'A' ... 'A' 'A' 'A' 'A' V_i (Timestamp) float64 -1e+05 -1e+05 ... -1e+05 -1e+05 M_i_eff_tbt_model (Timestamp) float64 -1.0 -1.0 -1.0 ... -1.0 -1.0 -1.0 Phi_sc (Timestamp) float64 -2.264 -2.267 ... -1.901 -1.919 Longitude (Timestamp) float64 -95.37 -95.37 -95.37 ... 81.23 81.23 Longitude_GD (Timestamp) float64 -95.37 -95.37 -95.37 ... 81.23 81.23 ... ... OrbitDirection (Timestamp) int8 1 1 1 1 1 1 1 1 ... -1 -1 -1 -1 -1 -1 -1 N_i_err (Timestamp) float64 -1.0 -1.0 -1.0 ... -1.0 -1.0 -1.0 V_i_Flags (Timestamp) uint32 65537 65537 65537 ... 65537 65537 OrbitNumber (Timestamp) int32 11819 11819 11819 ... 11834 11834 11834 M_i_eff_Flags (Timestamp) uint32 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 V_i_err (Timestamp) float64 -1.0 -1.0 -1.0 ... -1.0 -1.0 -1.0 Attributes: (3)
An initial preview of the data:
fig, axes = plt.subplots(nrows=4, sharex=True, figsize=(10, 7))
axes_r = [ax.twinx() for ax in axes]
# Plot quantity (left axis) and error (right axis) for each quantity
ds.plot.scatter(x="Timestamp", y="M_i_eff", ax=axes[0], s=1, linewidths=0)
ds.plot.scatter(x="Timestamp", y="M_i_eff_err", ax=axes_r[0], s=0.1, color="tab:orange")
ds.plot.scatter(x="Timestamp", y="V_i", ax=axes[1], s=1, linewidths=0)
ds.plot.scatter(x="Timestamp", y="V_i_err", ax=axes_r[1], s=0.1, color="tab:orange")
ds.plot.scatter(x="Timestamp", y="N_i", ax=axes[2], s=1, linewidths=0)
ds.plot.scatter(x="Timestamp", y="N_i_err", ax=axes_r[2], s=0.1, color="tab:orange")
ds.plot.scatter(x="Timestamp", y="T_e", ax=axes[3], s=1, linewidths=0)
fig.subplots_adjust(hspace=0)
# Add legend to identify each side
blue = mpl.patches.Patch(color="tab:blue", label="Quantities")
orange = mpl.patches.Patch(color="tab:orange", label="Errors")
axes[0].legend(handles=[blue, orange])
# # Generate additional ticklabels for x-axis
# Use time xticks to get dataset vars at those xticks
locx = axes[-1].get_xticks()
times = mpl.dates.num2date(locx)
times = [t.replace(tzinfo=None) for t in times]
_ds_xticks = ds.reindex({"Timestamp": times}, method="nearest")
# Build ticklabels from dataset vars
xticklabels = np.stack([
_ds_xticks["Timestamp"].dt.strftime("%H:%M").values,
np.round(_ds_xticks["Latitude"].values, 2).astype(str),
np.round(_ds_xticks["Longitude"].values, 2).astype(str),
])
xticklabels = ["\n".join(row) for row in xticklabels.T]
# Add labels to first xtick
_xt0 = xticklabels[0].split("\n")
xticklabels[0] = f"Time: {_xt0[0]}\nLat: {_xt0[1]}\nLon: {_xt0[2]}"
axes[-1].set_xticks(axes[-1].get_xticks())
axes[-1].set_xticklabels(xticklabels)
axes[-1].set_xlabel("")
# Adjust title
sources = "\n".join([i for i in ds.attrs["Sources"] if "IDM" in i])
title = "".join([
f"Swarm {ds['Spacecraft'].data[0]} ion effective mass and drift, ",
ds["Timestamp"].dt.date.data[0].isoformat(),
f"\n{sources}"
])
fig.suptitle(title);
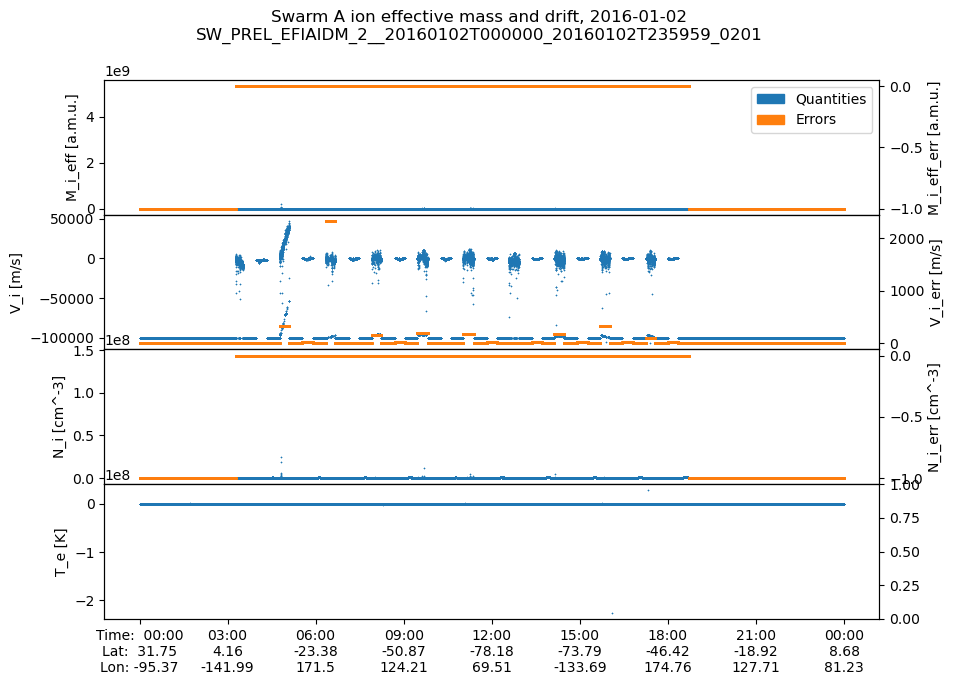