MAGxLR_1B (Magnetic field 1Hz)#
Abstract: Access to the low rate (1Hz) magnetic data (level 1b product), together with geomagnetic model evaluations (level 2 products).
%load_ext watermark
%watermark -i -v -p viresclient,pandas,xarray,matplotlib
Python implementation: CPython
Python version : 3.11.6
IPython version : 8.18.0
viresclient: 0.12.0
pandas : 2.1.3
xarray : 2023.12.0
matplotlib : 3.8.2
from viresclient import SwarmRequest
import datetime as dt
import matplotlib.pyplot as plt
request = SwarmRequest()
Product information#
This is one of the main products from Swarm - the 1Hz measurements of the magnetic field vector (B_NEC
) and total intensity (F
). These are derived from the Vector Field Magnetometer (VFM) and Absolute Scalar Magnetomer (ASM).
Documentation:
Measurements are available through VirES as part of collections with names containing MAGx_LR
, for each Swarm spacecraft:
request.available_collections("MAG", details=False)
{'MAG': ['SW_OPER_MAGA_LR_1B',
'SW_OPER_MAGB_LR_1B',
'SW_OPER_MAGC_LR_1B',
'SW_FAST_MAGA_LR_1B',
'SW_FAST_MAGB_LR_1B',
'SW_FAST_MAGC_LR_1B']}
The measurements can be used together with geomagnetic model evaluations as shall be shown below.
Check what “MAG” data variables are available#
request.available_measurements("MAG")
['F',
'dF_Sun',
'dF_AOCS',
'dF_other',
'F_error',
'B_VFM',
'B_NEC',
'dB_Sun',
'dB_AOCS',
'dB_other',
'B_error',
'q_NEC_CRF',
'Att_error',
'Flags_F',
'Flags_B',
'Flags_q',
'Flags_Platform',
'ASM_Freq_Dev']
Check the names of available models#
request.available_models(details=False)
['IGRF',
'LCS-1',
'MF7',
'CHAOS-Core',
'CHAOS-Static',
'CHAOS-MMA-Primary',
'CHAOS-MMA-Secondary',
'MCO_SHA_2C',
'MCO_SHA_2D',
'MLI_SHA_2C',
'MLI_SHA_2D',
'MLI_SHA_2E',
'MMA_SHA_2C-Primary',
'MMA_SHA_2C-Secondary',
'MMA_SHA_2F-Primary',
'MMA_SHA_2F-Secondary',
'MIO_SHA_2C-Primary',
'MIO_SHA_2C-Secondary',
'MIO_SHA_2D-Primary',
'MIO_SHA_2D-Secondary',
'AMPS',
'MCO_SHA_2X',
'CHAOS',
'CHAOS-MMA',
'MMA_SHA_2C',
'MMA_SHA_2F',
'MIO_SHA_2C',
'MIO_SHA_2D',
'SwarmCI']
Fetch some MAG data and models#
We can fetch the data and the model predictions (evaluated on demand) at the same time. We can also subsample the data - here we subsample it to 10-seconds by specifying the “PT10S” sampling_step
.
request.set_collection("SW_OPER_MAGA_LR_1B")
request.set_products(
measurements=["F", "B_NEC"],
models=["CHAOS-Core", "MCO_SHA_2D"],
sampling_step="PT10S"
)
data = request.get_between(
# 2014-01-01 00:00:00
start_time = dt.datetime(2014,1,1, 0),
# 2014-01-01 01:00:00
end_time = dt.datetime(2014,1,1, 1)
)
See a list of the source files#
data.sources
['SW_OPER_MAGA_LR_1B_20140101T000000_20140101T235959_0602_MDR_MAG_LR',
'SW_OPER_MCO_SHA_2D_20131126T000000_20180101T000000_0401',
'SW_OPER_MCO_SHA_2X_19970101T000000_20240807T235959_0718']
Load as a pandas dataframe#
Use expand=True
to extract vectors (B_NEC…) as separate columns (…_N, …_E, …_C)
df = data.as_dataframe(expand=True)
df.head()
F_CHAOS-Core | Latitude | Radius | F_MCO_SHA_2D | Longitude | F | Spacecraft | B_NEC_N | B_NEC_E | B_NEC_C | B_NEC_MCO_SHA_2D_N | B_NEC_MCO_SHA_2D_E | B_NEC_MCO_SHA_2D_C | B_NEC_CHAOS-Core_N | B_NEC_CHAOS-Core_E | B_NEC_CHAOS-Core_C | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | ||||||||||||||||
2014-01-01 00:00:00 | 22874.133948 | -1.228937 | 6878309.29 | 22874.210752 | -14.116675 | 22867.4381 | A | 20103.5295 | -4126.0959 | -10086.8832 | 20113.623744 | -4127.463817 | -10081.453262 | 20112.944066 | -4126.931232 | -10082.852943 |
2014-01-01 00:00:10 | 22820.867039 | -1.862520 | 6878381.24 | 22820.940668 | -14.131425 | 22814.4502 | A | 19815.0632 | -4160.8621 | -10514.3566 | 19825.161689 | -4163.127409 | -10508.409355 | 19824.453390 | -4162.576127 | -10509.804002 |
2014-01-01 00:00:20 | 22769.294251 | -2.496089 | 6878452.12 | 22769.368411 | -14.146156 | 22763.1399 | A | 19523.4409 | -4195.1070 | -10926.9487 | 19533.553819 | -4197.528908 | -10920.859129 | 19532.814808 | -4196.959308 | -10922.245142 |
2014-01-01 00:00:30 | 22719.159330 | -3.129643 | 6878521.94 | 22719.237483 | -14.160861 | 22713.2484 | A | 19229.2127 | -4228.3665 | -11324.7764 | 19239.343461 | -4230.601678 | -11318.720089 | 19238.572249 | -4230.014182 | -11320.093572 |
2014-01-01 00:00:40 | 22670.218653 | -3.763183 | 6878590.68 | 22670.303932 | -14.175535 | 22664.5952 | A | 18932.8424 | -4260.6440 | -11708.0879 | 18943.075099 | -4262.280487 | -11701.946471 | 18942.270857 | -4261.675566 | -11703.303377 |
… or as an xarray dataset:#
ds = data.as_xarray()
ds
<xarray.Dataset> Dimensions: (Timestamp: 360, NEC: 3) Coordinates: * Timestamp (Timestamp) datetime64[ns] 2014-01-01 ... 2014-01-01T00... * NEC (NEC) <U1 'N' 'E' 'C' Data variables: Spacecraft (Timestamp) object 'A' 'A' 'A' 'A' 'A' ... 'A' 'A' 'A' 'A' B_NEC_CHAOS-Core (Timestamp, NEC) float64 2.011e+04 ... 3.557e+04 F_CHAOS-Core (Timestamp) float64 2.287e+04 2.282e+04 ... 4.02e+04 Latitude (Timestamp) float64 -1.229 -1.863 -2.496 ... 48.14 48.77 Radius (Timestamp) float64 6.878e+06 6.878e+06 ... 6.868e+06 F_MCO_SHA_2D (Timestamp) float64 2.287e+04 2.282e+04 ... 4.021e+04 Longitude (Timestamp) float64 -14.12 -14.13 -14.15 ... 153.6 153.6 B_NEC (Timestamp, NEC) float64 2.01e+04 -4.126e+03 ... 3.558e+04 B_NEC_MCO_SHA_2D (Timestamp, NEC) float64 2.011e+04 ... 3.557e+04 F (Timestamp) float64 2.287e+04 2.281e+04 ... 4.021e+04 Attributes: Sources: ['SW_OPER_MAGA_LR_1B_20140101T000000_20140101T235959_060... MagneticModels: ["CHAOS-Core = 'CHAOS-Core'(max_degree=20,min_degree=1)"... AppliedFilters: []
Fetch the residuals directly#
Adding residuals=True
to .set_products()
will instead directly evaluate and return all data-model residuals
request = SwarmRequest()
request.set_collection("SW_OPER_MAGA_LR_1B")
request.set_products(
measurements=["F", "B_NEC"],
models=["CHAOS-Core", "MCO_SHA_2D"],
residuals=True,
sampling_step="PT10S"
)
data = request.get_between(
start_time = dt.datetime(2014,1,1, 0),
end_time = dt.datetime(2014,1,1, 1)
)
df = data.as_dataframe(expand=True)
df.head()
F_res_MCO_SHA_2D | Latitude | F_res_CHAOS-Core | Radius | Longitude | Spacecraft | B_NEC_res_MCO_SHA_2D_N | B_NEC_res_MCO_SHA_2D_E | B_NEC_res_MCO_SHA_2D_C | B_NEC_res_CHAOS-Core_N | B_NEC_res_CHAOS-Core_E | B_NEC_res_CHAOS-Core_C | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | ||||||||||||
2014-01-01 00:00:00 | -6.772652 | -1.228937 | -6.695848 | 6878309.29 | -14.116675 | A | -10.094244 | 1.367917 | -5.429938 | -9.414566 | 0.835332 | -4.030257 |
2014-01-01 00:00:10 | -6.490468 | -1.862520 | -6.416839 | 6878381.24 | -14.131425 | A | -10.098489 | 2.265309 | -5.947245 | -9.390190 | 1.714027 | -4.552598 |
2014-01-01 00:00:20 | -6.228511 | -2.496089 | -6.154351 | 6878452.12 | -14.146156 | A | -10.112919 | 2.421908 | -6.089571 | -9.373908 | 1.852308 | -4.703558 |
2014-01-01 00:00:30 | -5.989083 | -3.129643 | -5.910930 | 6878521.94 | -14.160861 | A | -10.130761 | 2.235178 | -6.056311 | -9.359549 | 1.647682 | -4.682828 |
2014-01-01 00:00:40 | -5.708732 | -3.763183 | -5.623453 | 6878590.68 | -14.175535 | A | -10.232699 | 1.636487 | -6.141429 | -9.428457 | 1.031566 | -4.784523 |
Plot the scalar residuals#
… using the pandas method:#
ax = df.plot(
y=["F_res_CHAOS-Core", "F_res_MCO_SHA_2D"],
figsize=(15,5),
grid=True
)
ax.set_xlabel("Timestamp")
ax.set_ylabel("[nT]");
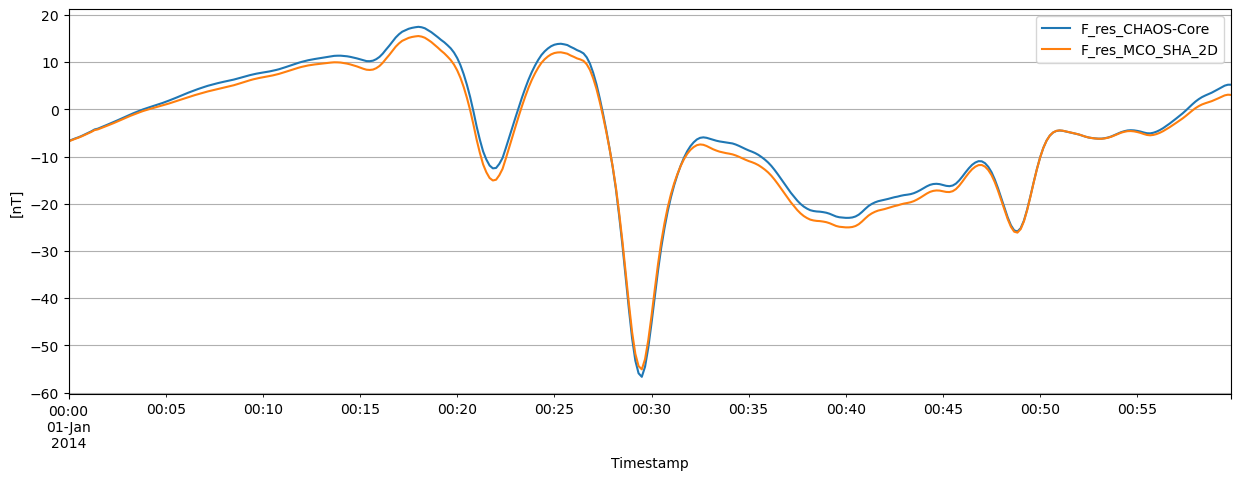
… using matplotlib interface#
NB: we are doing plt.plot(x, y)
with x
as df.index
(the time-based index of df), and y
as df[".."]
plt.figure(figsize=(15,5))
plt.plot(
df.index,
df["F_res_CHAOS-Core"],
label="F_res_CHAOS-Core"
)
plt.plot(
df.index,
df["F_res_MCO_SHA_2D"],
label="F_res_MCO_SHA_2D"
)
plt.xlabel("Timestamp")
plt.ylabel("[nT]")
plt.grid()
plt.legend();
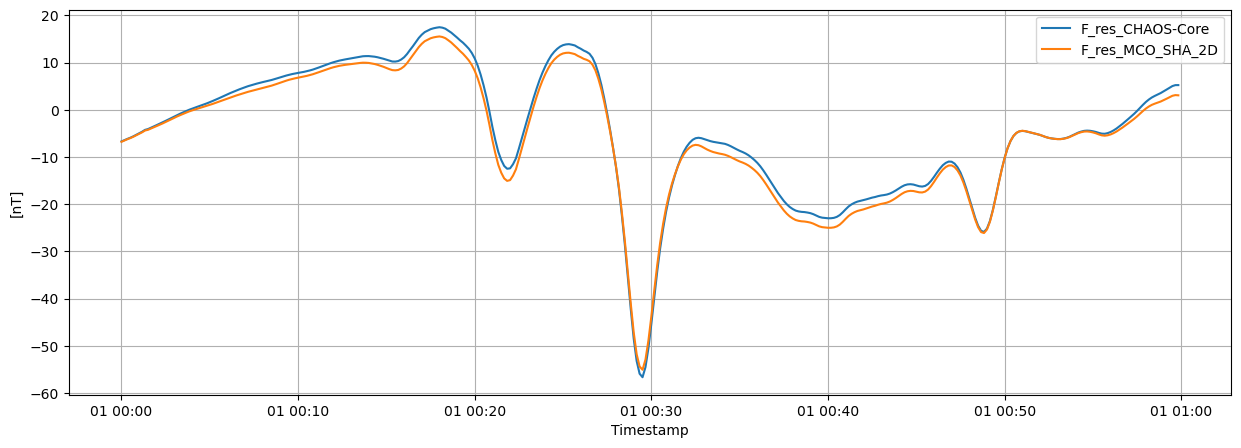
… using matplotlib interface (Object Oriented style)#
This is the recommended route for making more complicated figures
fig, ax = plt.subplots(figsize=(15,5))
ax.plot(
df.index,
df["F_res_CHAOS-Core"],
label="F_res_CHAOS-Core"
)
ax.plot(
df.index,
df["F_res_MCO_SHA_2D"],
label="F_res_MCO_SHA_2D"
)
ax.set_xlabel("Timestamp")
ax.set_ylabel("[nT]")
ax.grid()
ax.legend();
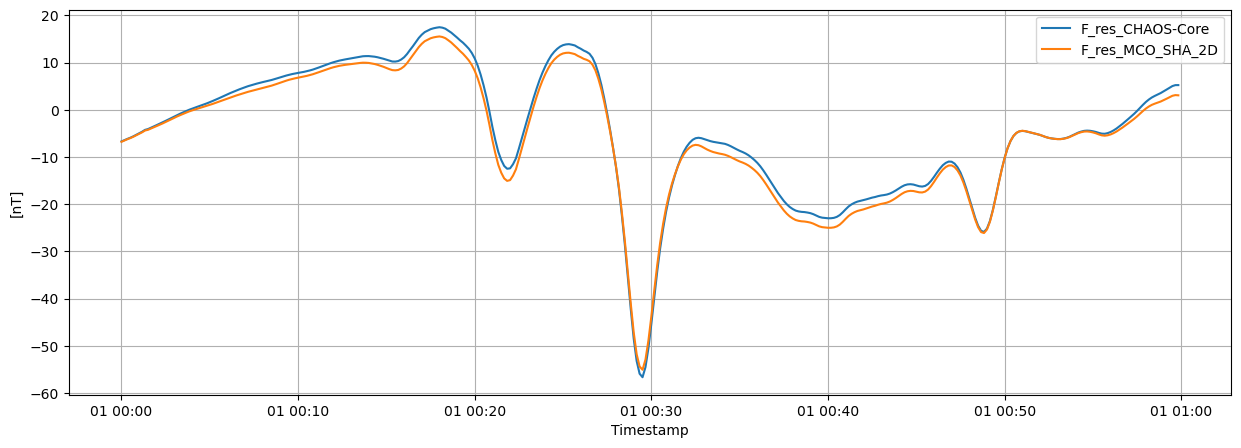
Plot the vector components#
fig, axes = plt.subplots(nrows=3, ncols=1, figsize=(15,10), sharex=True)
for component, ax in zip("NEC", axes):
for model_name in ("CHAOS-Core", "MCO_SHA_2D"):
ax.plot(
df.index,
df[f"B_NEC_res_{model_name}_{component}"],
label=model_name
)
ax.set_ylabel(f"{component}\n[nT]")
ax.legend()
axes[0].set_title("Residuals to models (NEC components)")
axes[2].set_xlabel("Timestamp");
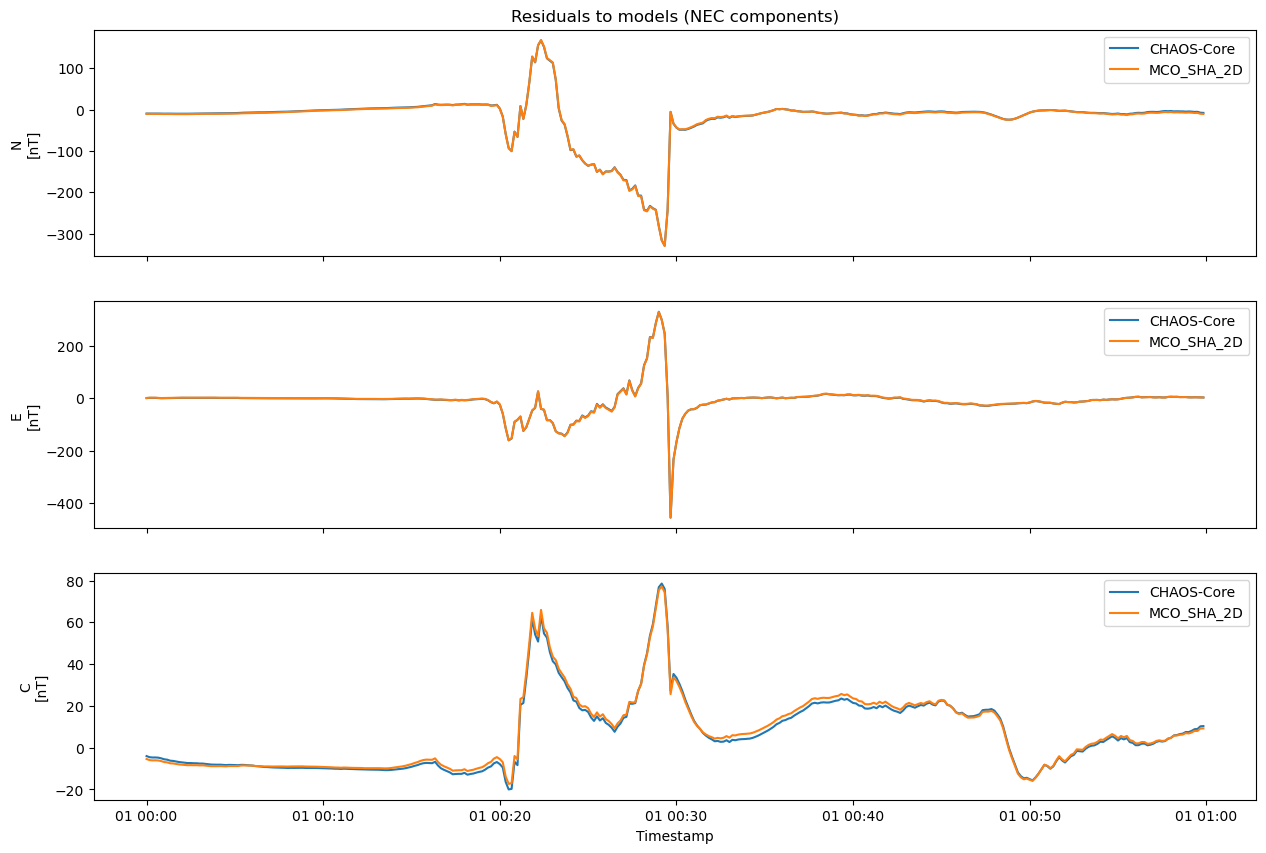
Similar plotting, using the data via xarray instead#
xarray provides a more sophisticated data structure that is more suitable for the complex vector data we are accessing, together with nice stuff like unit and other metadata support. Unfortunately due to the extra complexity, this can make it difficult to use right away.
ds = data.as_xarray()
ds
<xarray.Dataset> Dimensions: (Timestamp: 360, NEC: 3) Coordinates: * Timestamp (Timestamp) datetime64[ns] 2014-01-01 ... 2014-01-0... * NEC (NEC) <U1 'N' 'E' 'C' Data variables: Spacecraft (Timestamp) object 'A' 'A' 'A' 'A' ... 'A' 'A' 'A' 'A' F_res_MCO_SHA_2D (Timestamp) float64 -6.773 -6.49 ... 3.122 3.077 B_NEC_res_CHAOS-Core (Timestamp, NEC) float64 -9.415 0.8353 ... 3.148 10.32 Latitude (Timestamp) float64 -1.229 -1.863 ... 48.14 48.77 F_res_CHAOS-Core (Timestamp) float64 -6.696 -6.417 ... 5.238 5.241 Radius (Timestamp) float64 6.878e+06 6.878e+06 ... 6.868e+06 Longitude (Timestamp) float64 -14.12 -14.13 ... 153.6 153.6 B_NEC_res_MCO_SHA_2D (Timestamp, NEC) float64 -10.09 1.368 ... 3.074 9.198 Attributes: Sources: ['SW_OPER_MAGA_LR_1B_20140101T000000_20140101T235959_060... MagneticModels: ["CHAOS-Core = 'CHAOS-Core'(max_degree=20,min_degree=1)"... AppliedFilters: []
fig, axes = plt.subplots(nrows=3, ncols=1, figsize=(15,10), sharex=True)
for i, ax in enumerate(axes):
for model_name in ("CHAOS-Core", "MCO_SHA_2D"):
ax.plot(
ds["Timestamp"],
ds[f"B_NEC_res_{model_name}"][:, i],
label=model_name
)
ax.set_ylabel("NEC"[i] + " [nT]")
ax.legend()
axes[0].set_title("Residuals to models (NEC components)")
axes[2].set_xlabel("Timestamp");
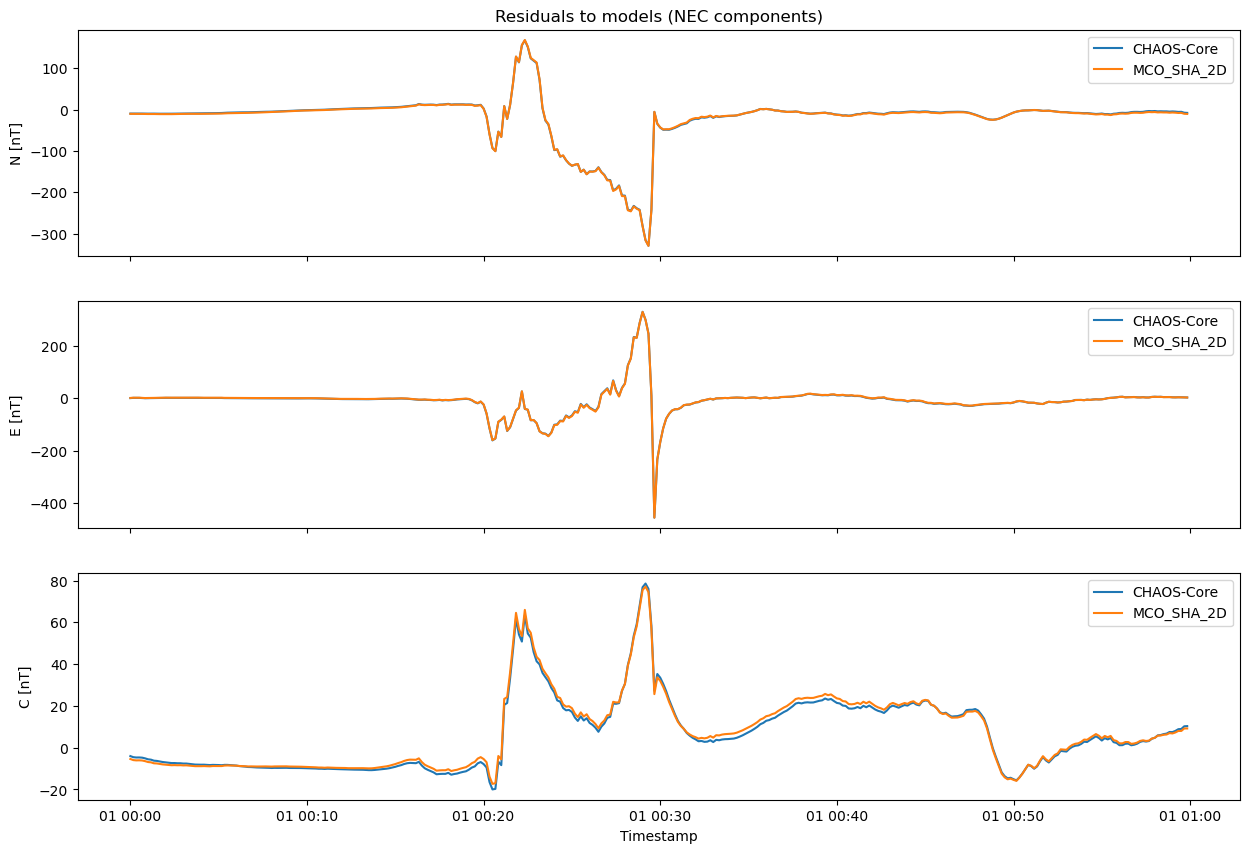
Note that xarray also allows convenient direct plotting like:
ds["B_NEC_res_CHAOS-Core"].plot.line(x="Timestamp");
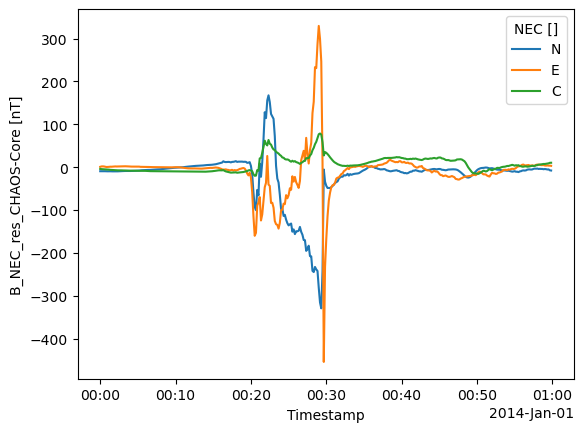
Access multiple MAG datasets simultaneously#
It is possible to fetch data from multiple collections simultaneously. Here we fetch the measurements from Swarm Alpha and Bravo. In the returned data, you can differentiate between them using the “Spacecraft” column.
request = SwarmRequest()
request.set_collection("SW_OPER_MAGA_LR_1B", "SW_OPER_MAGC_LR_1B")
request.set_products(
measurements=["F", "B_NEC"],
models=["CHAOS-Core",],
residuals=True,
sampling_step="PT10S"
)
data = request.get_between(
start_time = dt.datetime(2014,1,1, 0),
end_time = dt.datetime(2014,1,1, 1)
)
df = data.as_dataframe(expand=True)
df.head()
Latitude | F_res_CHAOS-Core | Radius | Longitude | Spacecraft | B_NEC_res_CHAOS-Core_N | B_NEC_res_CHAOS-Core_E | B_NEC_res_CHAOS-Core_C | |
---|---|---|---|---|---|---|---|---|
Timestamp | ||||||||
2014-01-01 00:00:00 | -1.228937 | -6.695848 | 6878309.29 | -14.116675 | A | -9.414566 | 0.835332 | -4.030257 |
2014-01-01 00:00:10 | -1.862520 | -6.416839 | 6878381.24 | -14.131425 | A | -9.390190 | 1.714027 | -4.552598 |
2014-01-01 00:00:20 | -2.496089 | -6.154351 | 6878452.12 | -14.146156 | A | -9.373908 | 1.852308 | -4.703558 |
2014-01-01 00:00:30 | -3.129643 | -5.910930 | 6878521.94 | -14.160861 | A | -9.359549 | 1.647682 | -4.682828 |
2014-01-01 00:00:40 | -3.763183 | -5.623453 | 6878590.68 | -14.175535 | A | -9.428457 | 1.031566 | -4.784523 |
df[df["Spacecraft"] == "A"].head()
Latitude | F_res_CHAOS-Core | Radius | Longitude | Spacecraft | B_NEC_res_CHAOS-Core_N | B_NEC_res_CHAOS-Core_E | B_NEC_res_CHAOS-Core_C | |
---|---|---|---|---|---|---|---|---|
Timestamp | ||||||||
2014-01-01 00:00:00 | -1.228937 | -6.695848 | 6878309.29 | -14.116675 | A | -9.414566 | 0.835332 | -4.030257 |
2014-01-01 00:00:10 | -1.862520 | -6.416839 | 6878381.24 | -14.131425 | A | -9.390190 | 1.714027 | -4.552598 |
2014-01-01 00:00:20 | -2.496089 | -6.154351 | 6878452.12 | -14.146156 | A | -9.373908 | 1.852308 | -4.703558 |
2014-01-01 00:00:30 | -3.129643 | -5.910930 | 6878521.94 | -14.160861 | A | -9.359549 | 1.647682 | -4.682828 |
2014-01-01 00:00:40 | -3.763183 | -5.623453 | 6878590.68 | -14.175535 | A | -9.428457 | 1.031566 | -4.784523 |
df[df["Spacecraft"] == "C"].head()
Latitude | F_res_CHAOS-Core | Radius | Longitude | Spacecraft | B_NEC_res_CHAOS-Core_N | B_NEC_res_CHAOS-Core_E | B_NEC_res_CHAOS-Core_C | |
---|---|---|---|---|---|---|---|---|
Timestamp | ||||||||
2014-01-01 00:00:00 | 5.908083 | -10.098784 | 6877665.96 | -14.420068 | C | -10.085120 | 1.993727 | -0.316837 |
2014-01-01 00:00:10 | 5.274386 | -9.724007 | 6877747.64 | -14.434576 | C | -9.854962 | 2.075957 | -0.815382 |
2014-01-01 00:00:20 | 4.640702 | -9.431278 | 6877828.37 | -14.449141 | C | -9.761243 | 1.978650 | -1.273802 |
2014-01-01 00:00:30 | 4.007031 | -9.215576 | 6877908.13 | -14.463755 | C | -9.880403 | 1.576165 | -1.887636 |
2014-01-01 00:00:40 | 3.373371 | -8.991461 | 6877986.91 | -14.478411 | C | -9.981207 | 1.066904 | -2.313399 |
… or using xarray#
ds = data.as_xarray()
ds.where(ds["Spacecraft"] == "A", drop=True)
<xarray.Dataset> Dimensions: (Timestamp: 360, NEC: 3) Coordinates: * Timestamp (Timestamp) datetime64[ns] 2014-01-01 ... 2014-01-0... * NEC (NEC) <U1 'N' 'E' 'C' Data variables: Spacecraft (Timestamp) object 'A' 'A' 'A' 'A' ... 'A' 'A' 'A' 'A' B_NEC_res_CHAOS-Core (Timestamp, NEC) float64 -9.415 0.8353 ... 3.148 10.32 Latitude (Timestamp) float64 -1.229 -1.863 ... 48.14 48.77 F_res_CHAOS-Core (Timestamp) float64 -6.696 -6.417 ... 5.238 5.241 Radius (Timestamp) float64 6.878e+06 6.878e+06 ... 6.868e+06 Longitude (Timestamp) float64 -14.12 -14.13 ... 153.6 153.6 Attributes: Sources: ['SW_OPER_MAGA_LR_1B_20140101T000000_20140101T235959_060... MagneticModels: ["CHAOS-Core = 'CHAOS-Core'(max_degree=20,min_degree=1)"] AppliedFilters: []