TECxTMS_2F (Total electron content)#
Abstract: Access to the total electric contents (level 2 product).
%load_ext watermark
%watermark -i -v -p viresclient,pandas,xarray,matplotlib
Python implementation: CPython
Python version : 3.11.6
IPython version : 8.18.0
viresclient: 0.12.3
pandas : 2.1.3
xarray : 2023.12.0
matplotlib : 3.8.2
from viresclient import SwarmRequest
import datetime as dt
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
request = SwarmRequest()
TECxTMS_2F product information#
Derived total electron content (TEC)
Documentation:
Check what “TEC” data variables are available#
request.available_collections("IPD", details=False)
{'IPD': ['SW_OPER_IPDAIRR_2F', 'SW_OPER_IPDBIRR_2F', 'SW_OPER_IPDCIRR_2F']}
request.available_measurements("TEC")
['GPS_Position',
'LEO_Position',
'PRN',
'L1',
'L2',
'P1',
'P2',
'S1',
'S2',
'Elevation_Angle',
'Absolute_VTEC',
'Absolute_STEC',
'Relative_STEC',
'Relative_STEC_RMS',
'DCB',
'DCB_Error']
Fetch one day of TEC data#
request.set_collection("SW_OPER_TECATMS_2F")
request.set_products(measurements=request.available_measurements("TEC"))
data = request.get_between(dt.datetime(2014,1,1),
dt.datetime(2014,1,2))
Loading as pandas#
df = data.as_dataframe()
df.head()
Relative_STEC | S1 | DCB_Error | Radius | Latitude | GPS_Position | Elevation_Angle | Absolute_VTEC | LEO_Position | P2 | Spacecraft | DCB | L2 | S2 | Absolute_STEC | P1 | PRN | Longitude | Relative_STEC_RMS | L1 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | ||||||||||||||||||||
2014-01-01 00:00:04 | 24.041127 | 36.83 | 0.832346 | 6.878338e+06 | -1.482419 | [22448765.690377887, 5421379.431197803, -13409... | 39.683333 | 10.894886 | [6668214.552999999, -1677732.678, -177943.998] | 2.182914e+07 | A | -11.446853 | -6.261989e+06 | 36.83 | 16.429938 | 2.182913e+07 | 15 | -14.122572 | 0.555675 | -6.261986e+06 |
2014-01-01 00:00:04 | 22.754795 | 34.75 | 0.832346 | 6.878338e+06 | -1.482419 | [16113499.491062254, -16306172.004347403, -126... | 37.478137 | 10.461554 | [6668214.552999999, -1677732.678, -177943.998] | 2.135749e+07 | A | -11.446853 | -1.466568e+07 | 34.75 | 16.456409 | 2.135749e+07 | 18 | -14.122572 | 0.488000 | -1.466568e+07 |
2014-01-01 00:00:04 | 15.585271 | 30.90 | 0.832346 | 6.878338e+06 | -1.482419 | [10823457.339250825, -24014739.352816023, -248... | 24.681787 | 9.529846 | [6668214.552999999, -1677732.678, -177943.998] | 2.277638e+07 | A | -11.446853 | -5.455454e+06 | 30.90 | 20.434286 | 2.277638e+07 | 22 | -14.122572 | 1.313984 | -5.455452e+06 |
2014-01-01 00:00:04 | 52.291341 | 29.87 | 0.832346 | 6.878338e+06 | -1.482419 | [20631539.59055339, 13441368.439225309, 100505... | 24.647445 | 9.357587 | [6668214.552999999, -1677732.678, -177943.998] | 2.298847e+07 | A | -11.446853 | -3.402821e+06 | 29.87 | 20.085094 | 2.298846e+07 | 24 | -14.122572 | 0.899036 | -3.402816e+06 |
2014-01-01 00:00:04 | 52.672067 | 30.88 | 0.832346 | 6.878338e+06 | -1.482419 | [16637723.905075422, -10692759.977004562, 1761... | 30.753636 | 8.597559 | [6668214.552999999, -1677732.678, -177943.998] | 2.229900e+07 | A | -11.446853 | -2.285986e+06 | 30.88 | 15.676947 | 2.229899e+07 | 25 | -14.122572 | 0.546729 | -2.285981e+06 |
NB: The time interval is not always the same:
times = df.index
np.unique(np.sort(np.diff(times.to_pydatetime())))
array([datetime.timedelta(0), datetime.timedelta(seconds=10)],
dtype=object)
len(df), 60*60*24
(49738, 86400)
Loading and plotting as xarray#
ds = data.as_xarray()
ds
<xarray.Dataset> Dimensions: (Timestamp: 49738, WGS84: 3) Coordinates: * Timestamp (Timestamp) datetime64[ns] 2014-01-01T00:00:04 ... 201... * WGS84 (WGS84) <U1 'X' 'Y' 'Z' Data variables: (12/20) Spacecraft (Timestamp) object 'A' 'A' 'A' 'A' ... 'A' 'A' 'A' 'A' Relative_STEC (Timestamp) float64 24.04 22.75 15.59 ... 16.92 26.51 S1 (Timestamp) float64 36.83 34.75 30.9 ... 23.03 37.73 37.5 DCB_Error (Timestamp) float64 0.8323 0.8323 ... 0.8323 0.8323 Radius (Timestamp) float64 6.878e+06 6.878e+06 ... 6.88e+06 Latitude (Timestamp) float64 -1.482 -1.482 -1.482 ... -81.7 -81.7 ... ... Absolute_STEC (Timestamp) float64 16.43 16.46 20.43 ... 10.77 10.78 P1 (Timestamp) float64 2.183e+07 2.136e+07 ... 2.171e+07 PRN (Timestamp) uint16 15 18 22 24 25 29 ... 7 15 16 18 21 26 Longitude (Timestamp) float64 -14.12 -14.12 -14.12 ... 1.559 1.559 Relative_STEC_RMS (Timestamp) float64 0.5557 0.488 1.314 ... 0.6458 3.041 L1 (Timestamp) float64 -6.262e+06 -1.467e+07 ... -3.41e+06 Attributes: Sources: ['SW_OPER_TECATMS_2F_20140101T000000_20140101T235959_0401'] MagneticModels: [] AppliedFilters: []
fig, axes = plt.subplots(nrows=2, ncols=1, figsize=(15,5), sharex=True)
ds["Absolute_VTEC"].plot.line(x="Timestamp", ax=axes[0])
ds["Absolute_STEC"].plot.line(x="Timestamp", ax=axes[1]);
fig.subplots_adjust(hspace=0)
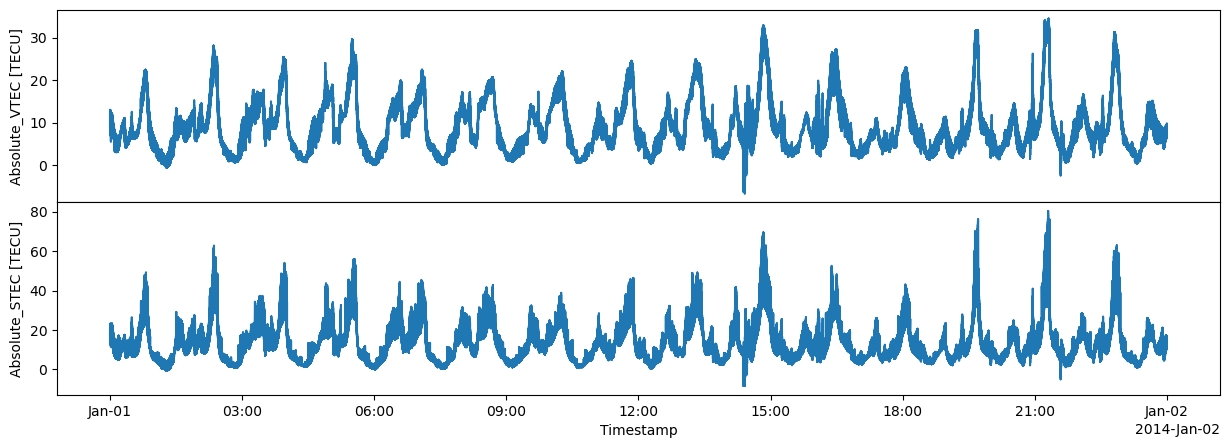